Within the realm of web development and the realm of API security, authentication assumes a pivotal role, serving as a safeguard to ensure that exclusively authorized entities gain access to delicate information. Basic authentication stands out as one of the most elementary facets of HTTP authentication, as it involves the transmission of user credentials alongside the client’s request to the server. This article is poised to embark on an exploration of the intricacies surrounding basic authentication, shedding light on its operational mechanics and offering insights into the optimal strategies for its secure implementation.
Understanding Basic Authentication in Depth
Basic authentication stands as one of the earliest and simplest methods integrated into the HTTP protocol to validate user credentials. It’s predominantly used to gain access to certain resources on a server that require a degree of restriction. This form of authentication leverages the act of sending both a username and password as part of the HTTP request, specifically in its header. These credentials are then processed through base64 encoding. While basic authentication is known for its ease of implementation, it doesn’t come without its fair share of limitations, especially in the realm of security. This comprehensive guide delves into the nuances of basic authentication and highlights its operational mechanism, potential risks, and more.
Features of Basic Authentication:
- Simplicity: Easy to set up and doesn’t require any additional libraries or plugins;
- Universality: It’s widely recognized across different platforms due to its integration with the HTTP protocol;
- Header-Based: Credentials are transmitted through the HTTP header after encoding.
However, to fully grasp the concept, one must understand the behind-the-scenes operation of this authentication mechanism.
Dynamics of Basic Authentication: A Step-by-Step Insight
Initial Client Request: When a client endeavors to access a server resource that necessitates authentication, the journey begins with the client sending a primary request.
- Server Response: In reaction to the client’s initial request, the server counters with a 401 Unauthorized status code. This is the server’s way of indicating that authentication is mandatory for access. Additionally, the server dispatches the ‘WWW-Authenticate’ header field as part of this response;
- Client’s Authenticated Response: On receiving the server’s feedback, the client crafts another request. This time, the request contains the ‘Authorization’ header field. The structure of this header is quite distinctive. It starts with the term ‘Basic’, succeeded by a space. What follows is a base64-encoded string which concatenates the username and password with a colon (username:password);
- Server’s Decoding Process: Once the server gets this authenticated request, it embarks on decoding the base64 string. Post-decoding, the server extracts both the username and password. The final step involves the server validating these credentials against its stored data to determine their legitimacy.
Safety Concerns & Recommendations for Basic Authentication:
- Potential Risks: Since basic authentication sends credentials in an encoded but not encrypted format, there’s a risk of interception by malicious entities;
- HTTPS Use: It’s paramount to use basic authentication in tandem with HTTPS to ensure an encrypted connection, adding an extra layer of security;
- Timed Sessions: Implement sessions that time out after inactivity, preventing prolonged access;
- Multifactor Authentication: Where possible, incorporate multifactor authentication to elevate security.
Generating a Robust Basic Authentication Token
Basic authentication tokens are foundational tools in the realm of web development, especially when it comes to API security. These tokens function as an access pass, allowing users to connect and interact with server resources. Here’s a thorough guide on how to create one:
- Concatenation Process: Begin by amalgamating the username and password using a colon;
- Example: Should the username be ‘admin’ and the password ‘12345’, the resultant string becomes ‘admin:12345’;
- Base64 Encoding: Once the string has been formed, encode this string using base64.
This transforms the readable string into a format that’s less easily interpreted, adding a level of security.
- Finalizing the Token: To cap it off, affix the encoded string with the prefix ‘Basic’, followed by a space;
- Resultant Format: If done correctly, the final token representation will resemble: ‘Basic YWRtaW46MTIzNDU=’;
- Helpful Tip: Always make sure to store these authentication tokens securely to prevent unwanted access and potential misuse.
Delving into the Strengths and Weaknesses of Basic Authentication
Basic authentication, while a time-tested method, comes with its own set of advantages and pitfalls. Familiarizing oneself with these can enable better-informed decisions.
Advantages:
- Effortless Implementation: Its simplicity is perhaps its biggest strength. With just a handful of code lines, one can have basic authentication up and running;
- Stateless Nature: This authentication method is stateless, meaning there’s no session maintained between the client and server. This:
- Reduces server memory usage;
- Offers straightforward scalability;
- Ensures no session data loss if a server crashes.
Limitations:
- Security Concerns Over HTTP: A major pitfall is the vulnerability over HTTP. Since the credentials transit in plain sight, it’s a goldmine for cybercriminals;
- Recommendation: Always opt for HTTPS, ensuring that communication lines are encrypted;
- Man-in-the-Middle (MitM) Attack Vulnerability: HTTPS does provide a shield, but it’s not impregnable. Basic authentication can fall prey to MitM attacks, especially if SSL/TLS certificates on the server side are compromised or not correctly validated;
- Insight: Regularly audit and update server certificates to mitigate this risk;
- Absence of a Logout Feature: A glaring limitation is the absence of a built-in logout mechanism. Since credentials tag along with every request, it can pose a security risk;
- Suggestion: Consider coupling basic authentication with other mechanisms or using a different method altogether for systems where logout functionality is crucial.
While basic authentication offers quick solutions, it’s imperative to weigh the pros and cons based on the specific needs and security requirements of a project.
Comprehensive Guide to Best Practices for Basic Authentication
When it comes to basic authentication, there are vital measures one must adopt to ensure a secure and effective communication system. As technology evolves, it’s more important than ever to be aware of potential vulnerabilities and how to counteract them. Here’s an in-depth look at the best practices to keep in mind:
Prioritize Encrypted Communications with HTTPS:
- HTTPS stands for Hypertext Transfer Protocol Secure, providing a secure version of HTTP;
- It ensures the data exchanged between a user’s browser and the server remains confidential through encryption;
- Without HTTPS, sensitive data can be intercepted or tampered with during transmission.
Ensure Valid SSL/TLS Certificates:
- SSL (Secure Sockets Layer) and its successor, TLS (Transport Layer Security), are protocols designed to secure data transmission;
- Always validate the server’s SSL/TLS certificate from the client side;
- Proper certificate validation can protect against man-in-the-middle attacks, where attackers secretly relay communication between two parties;
- Regularly update and renew certificates to maintain their authenticity.
Promote the Use of Strong, Unique Passwords:
- Strong passwords typically combine upper and lower case letters, numbers, and special characters;
- Avoid using easily guessable information like birthdays, names, or common words;
- Encourage users to change passwords regularly and avoid reusing old ones;
- Consider implementing password strength meters to guide users in creating robust passwords.
Guard Against Brute Force Attacks:
- Implement rate limiting to control the number of requests a user can make within a specified time frame;
- Incorporate account lockout mechanisms, which temporarily or permanently lock out a user after several failed login attempts;
- Monitor login attempts and flag suspicious activities.
Embrace Tokens for Session Management:
- In cases where session management is necessary, opt for token-based authentication;
- Tokens are dynamically generated strings of characters that authenticate and authorize users for specific sessions;
- They offer a more secure way to maintain user sessions without constantly requiring password input.
Exploring Alternatives to Basic Authentication
While basic authentication has its merits, there are several other authentication methods that offer increased security and flexibility. It’s wise to familiarize oneself with these alternatives, especially when building robust applications:
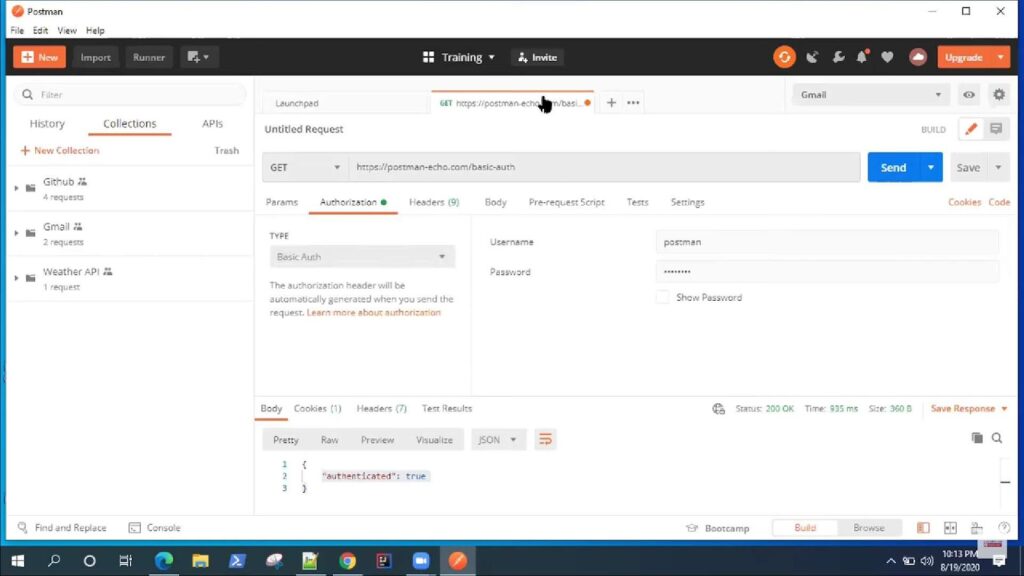
OAuth:
- An advanced authentication protocol that allows third-party access without exposing user credentials;
- Users grant limited access to their resources without sharing their passwords;
- Commonly used by major platforms like Google, Facebook, and Twitter for third-party integrations;
- Offers different “flows” for web apps, mobile apps, and desktop apps, catering to various use cases.
API Keys:
- These are unique identifier strings assigned to applications or users;
- They authenticate and authorize the client, allowing it to access specific resources or services;
- Keep API keys confidential, and avoid exposing them in client-side code to prevent misuse.
JWT (JSON Web Tokens):
- A compact and URL-safe token format;
- Carries claims between parties, which can be claims about an entity (typically the user) and potential claims about allowed actions;
- Self-contained and can store information like user details and permissions, reducing the need for database lookups;
- They are digitally signed, ensuring that the data hasn’t been altered during transmission.
By familiarizing oneself with these best practices and authentication alternatives, one can ensure a secure, effective, and user-friendly authentication experience.
Conclusion
Fundamental authentication represents a straightforward and stateless method of verifying user identities, often found to be appropriate for petite, low-security software implementations. Nonetheless, its inherent simplicity harbors vulnerabilities, necessitating a cautious approach when employing it. It is imperative to consistently employ HTTPS, rigorously validate SSL/TLS certificates, and contemplate employing more robust authentication alternatives for applications demanding elevated security thresholds. Adhering to industry best practices empowers developers to proactively minimize the inherent risks tied to basic authentication, thus fortifying the protective measures safeguarding their applications.